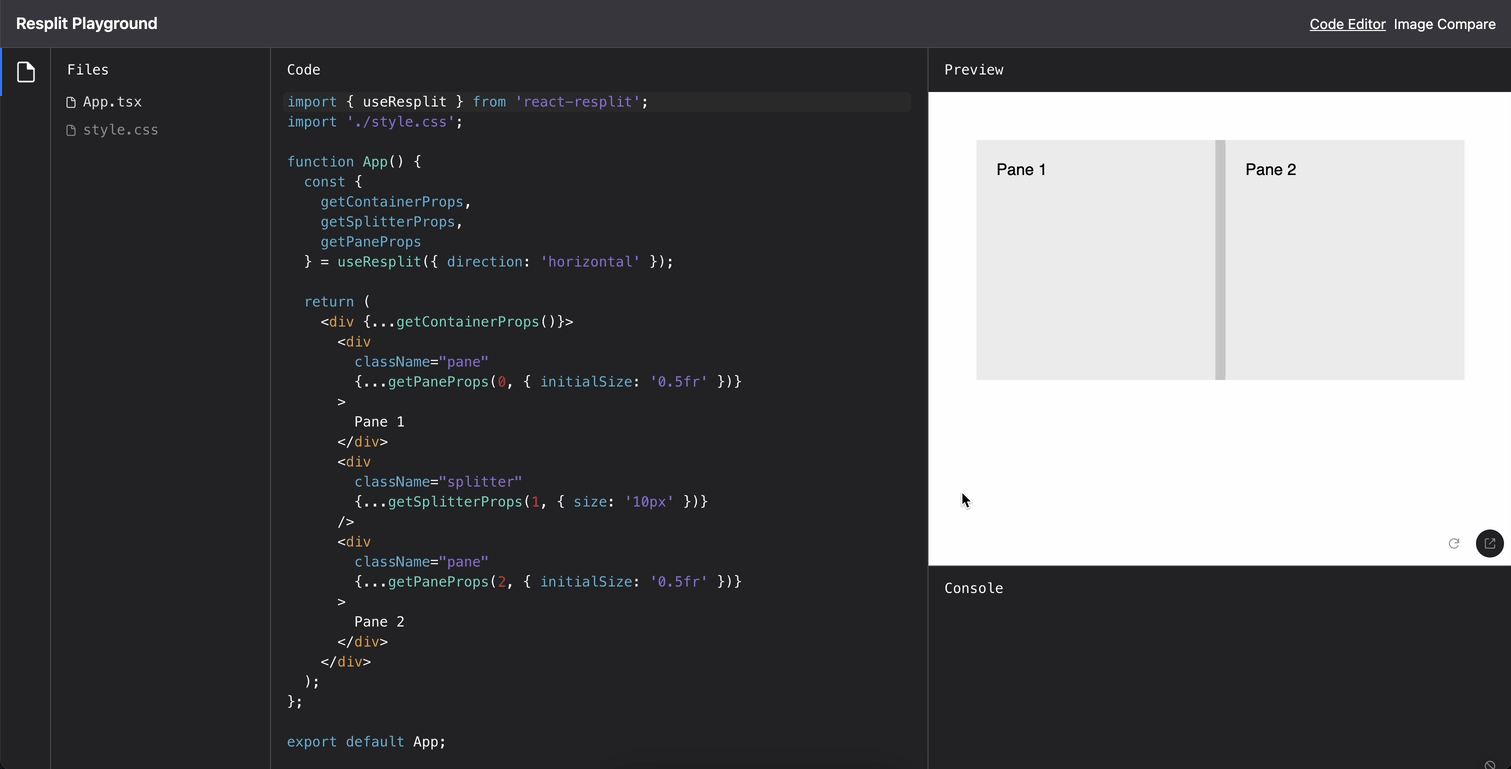
Resizable split pane layouts for React applications with react-resplit
Not long ago, I published react-resplit, an open source library for creating resizable split pane layouts in React applications.
Some of the key features that sets it apart from others in the space:
- Compound component API that works with any styling method
- Built with modern CSS, a grid-based layout and custom properties
- Works with any amount of panes in a vertical or horizontal layout
- Built following the Window Splitter pattern for accessibility and keyboard controls
Usage
It is pretty simple to use too, start by installing the package:
npm install react-resplit
Import Resplit
from react-resplit
and render the Root, Pane(s) and Splitter(s).
import { Resplit } from "react-resplit";
function App() {
return (
<Resplit.Root direction="horizontal">
<Resplit.Pane order={0} initialSize="0.5fr">
Pane 1
</Resplit.Pane>
<Resplit.Splitter order={1} size="10px" />
<Resplit.Pane order={2} initialSize="0.5fr">
Pane 2
</Resplit.Pane>
</Resplit.Root>
);
}
Styling
The Root, Splitter and Pane elements are all unstyled by default apart from a few styles that are necessary for the layout - this is intentional so that the library remains flexible.
Resplit will apply the correct cursor based on the direction
provided to the Root component.
As a basic example, you could provide a className prop to the Splitter elements and style them as a solid 10px divider.
<Resplit.Splitter className="splitter" order={0} size="10px" />
.splitter {
width: 100%;
height: 100%;
background: #ccc;
}
Accessibility
Resplit has been implemented using guidence from the Window Splitter pattern.
In addition to built-in accessibility considerations, you should also ensure that splitters are correctly labelled.
If the primary pane has a visible label, the aria-labelledby
attribute can be used.
<Resplit.Pane order={0}>
<h2 id="pane-1-label">Pane 1</h2>
</Resplit.Pane>
<Resplit.Splitter order={1} aria-labelledby="pane-1-label" />
Alternatively, if the pane does not have a visible label, the aria-label
attribute can be used.
<Resplit.Splitter order={1} aria-label="Pane 1" />
Interested in learning more?
For a more detailed look at the library, check out the GitHub repository.